Learn how to fix SEO warnings about the H1 title being too short on WooCommerce product pages. This is great when you want a more descriptive product title, but you don’t want to rename the underlying product. The example in this tutorial uses a product called “Puffer Jacket”. We want the H1 on the product page to read “Natural Goose Down Puffer Jacket”, but for WooCommerce to use “Puffer Jacket” everywhere else on the site.
We’ll use Advanced Custom Fields to create an “alternative h1 title” text field for our products (and posts). Then we’ll create a PHP snippet to hook a WordPress filter called the_title. This will override a product’s regular title if certain conditions are true.
Make sure you’ve got the latest version of the Advanced Custom Fields plugin installed. You’ll also need to be using a custom child theme so you can edit “functions.php”. If you’re not using a child theme, you can use a PHP code snippet manager plugin instead.
Configure an ACF field group
In the back-end of your site, go into ACF and create a field group called “H1 Title Override”. Open the field group and create a text field called “Alternative H1 title”. Make sure that the Field Name is exactly _alternative_h1_title
, because this is how we’ll refer to the field in our code. You can use anything you want for the Field Label.
Now create a Location Rule for each post type you want to use alternative titles for. We’re using “Product” and “Post” here:
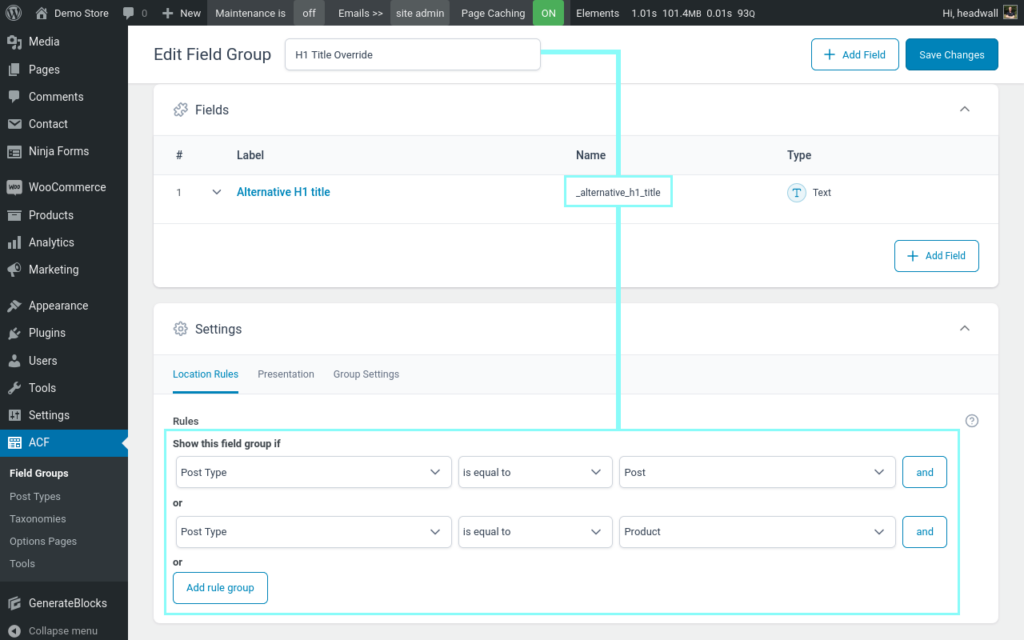
When you’ve saved your field group, edit a product that needs an alternative title. Find the “H1 Title Override” meta box, set the alternative title and save the product.
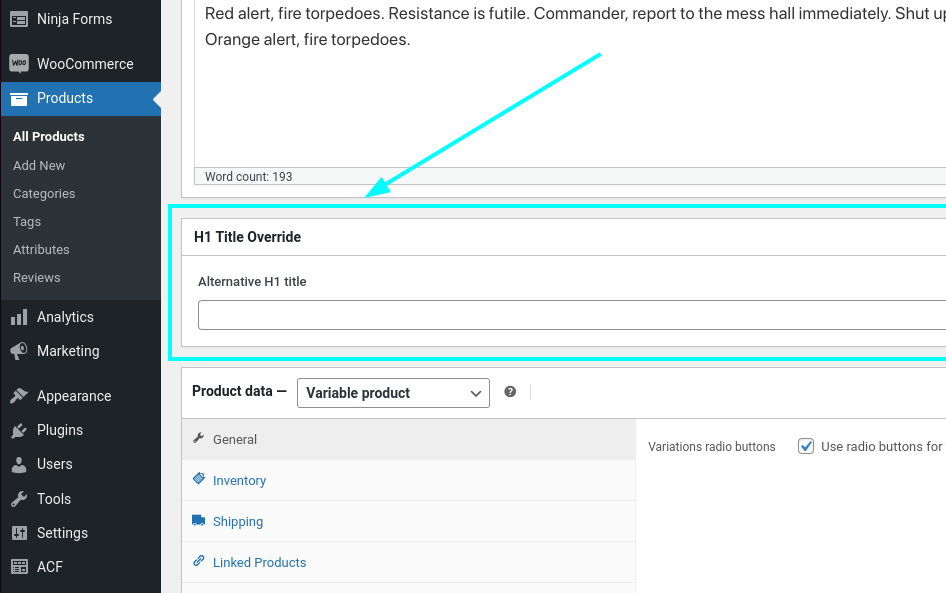
Override the title in PHP
In your child theme, create a file called wpt-alternative-h1.php and paste the following into it:
<?php /** * Headwall WP Tutorials : Alternative Singular H1 Title (WPTAST) * * https://wp-tutorials.tech/optimise-wordpress/fix-seo-warnings-about-h1-title-being-too-short/ * */ defined('WPINC') || die(); /** * Override the_title if a series of conditions are met. * * @param string $title The original/real title for the content * @param integer $post_id The post/product ID * * @return string */ function wptast_override_h1($post_title, $post_id) { if (is_admin() || wp_doing_ajax()) { // ... } elseif (!is_singular()) { // Don't change anything if we're on an archive page. } elseif (!is_main_query()) { // Don't change anything if we're not running as part of the page's main query. } elseif ($post_id != get_the_id()) { // Make sure we're on the correct post. } elseif (empty(($override_title = strval(get_post_meta($post_id, '_alternative_h1_title', true))))) { // Don't do anything if the alternative title is empty. } else { // Override the standard title now. $post_title = $override_title; } return $post_title; } add_filter('the_title', 'wptast_override_h1', 10, 2);
There’s not much to the code so it should be easy to follow. In short, the “the_title” filter is triggered each time WordPress wants to render a post’s title. That might be for the H1, it might be for a product card on an archive page, or it might be in the user’s cart items. So our function runs a few tests to make sure we’re on a single page (not an archive page) and we’re rendering the main content for that page (i.e. the product). We only override the title when all these conditions are met. You can adjust these tests if you want to change the behaviour – just add or remove your elseif(...){...}
conditions in the series of checks.
The important part is where we call get_post_meta($post_id, '_alternative_h1_title', true)
to fetch text for the alternative title.
Next, open your child theme’s functions.php file, add the following couple of lines and save it:
// Headwall WP Tutorials : Override the H1 title on simple single products/posts. require_once dirname(__FILE__) . '/wpt-alternative-h1.php';
Featured plugin
Deploy and test
Make sure you’ve added an alternative H1 title to one of your products and check that the regular product name is shown on the shop page. Then you can view the product page and verify the alternative title is showing correctly, like this:
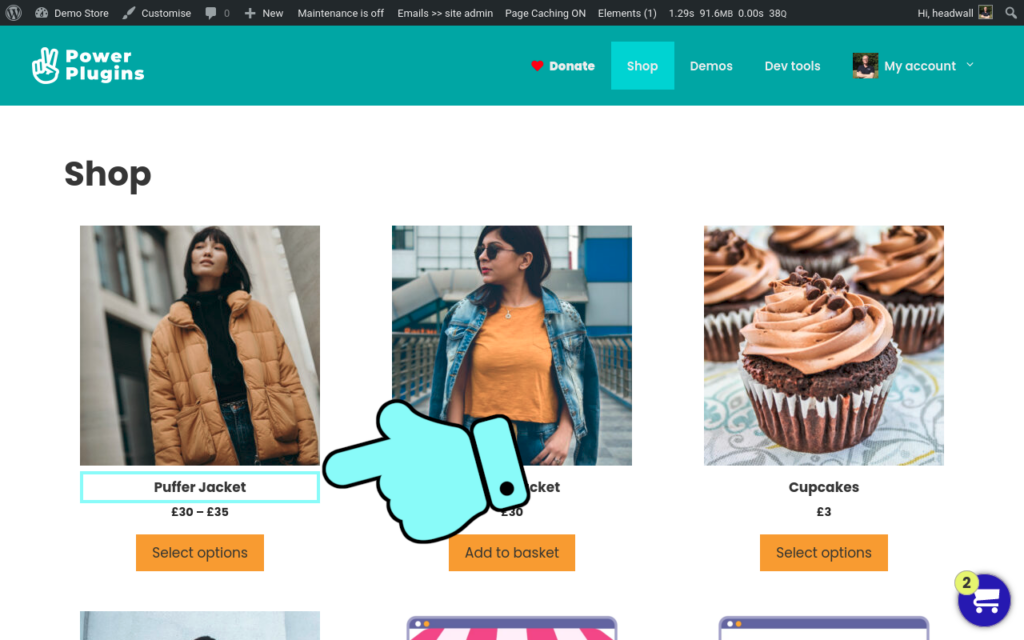
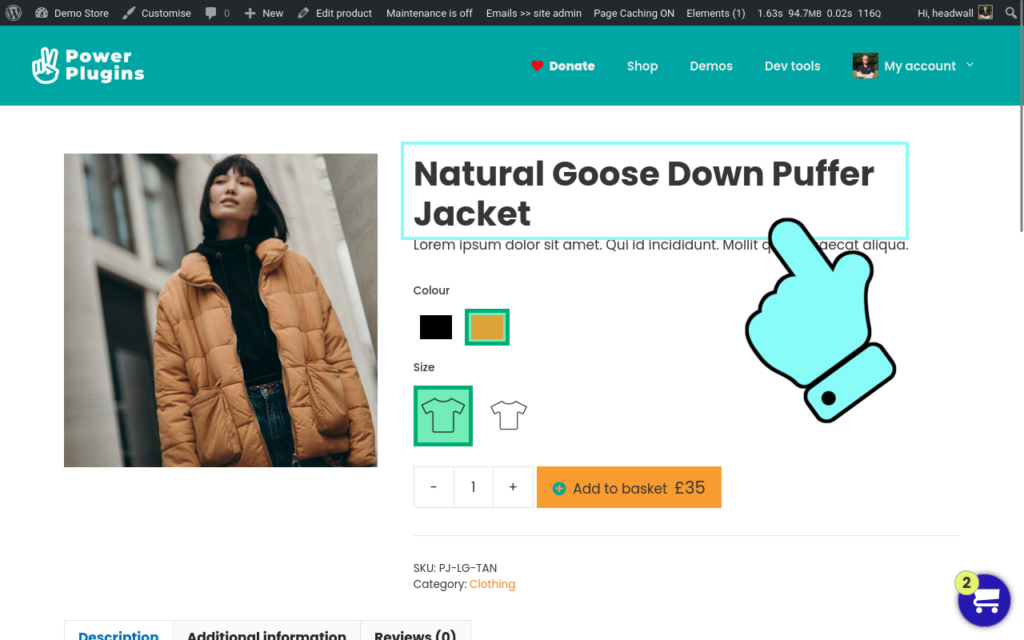
That’s it. A neat little hack that lets you add a more detailed H1 to your product pages, without making a mess of your shop archive/category pages 😎 👍