The standard WordPress jQuery version is 1.12.4 (in WordPress 5.5). If you run Google Page Speed over a fairly standard WordPress 5 page, you’ll see an error under Best Practices stating that the page, “Includes front-end JavaScript libraries with known security vulnerabilities”. It then goes on to reference jQuery 1.12.4 and says there are vulnerabilities associated with it.
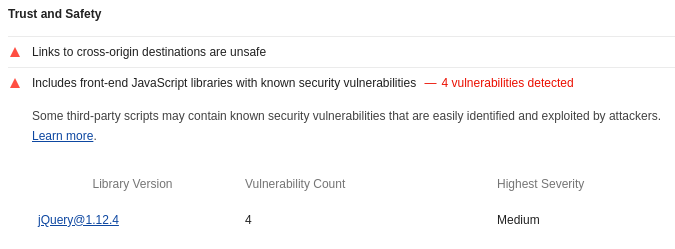
This is because WordPress 5.5 ships with jQuery 1.12.4 (at the time of writing – October 2020). Usually, it’s quite safe to upgrade to a later version of jQuery on a WordPress site, so…
Let’s Write the Code
Rather than just dump a bunch of code into our functions.php file, we’re going to add a bit of structure. This assumes that you’ve already created a custom child theme, which has its own functions.php file.
In your custom child theme’s folder, create a file called functions-jquery.php and paste the following code into it.
<?php // Block direct access. defined('WPINC') || die(); /** * Adjust the jQuery version. * * To use the stock WordPress jQuery do this fairly early in your theme's * functions.php: * * define('HW_USE_STOCK_JQUERY', true); * * If you want to pull jQuery from the CDN, even though you've got the local * self-hosted file available, you can do this: * * define('HW_USE_JQUERY_FROM_CDN', true); * * If you want to specify your own CDN, just define it in HW_JQUERY_CDN_SRC * and specify the version in HW_JQUERY_CDN_VERSION, like this: * * define('HW_JQUERY_CDN_VERSION', '3.5.1'); * define('HW_JQUERY_CDN_SRC', 'https://code.jquery.com/jquery-3.5.1.min.js'); * */ function hw_enqueue_scripts_update_jquery() { if (is_admin()) { return; } global $wp_scripts; if (array_key_exists('jquery-core', $wp_scripts->registered) && !defined('HW_USE_STOCK_JQUERY')) { $jquery_version = '3.5.1'; $jquery_file_name = sprintf('jq/jquery-%s.min.js', $jquery_version); $url_base = get_stylesheet_directory_uri(); $jquery_full_path = trailingslashit(dirname(__FILE__)) . $jquery_file_name; $jquery_src = null; if (is_readable($jquery_full_path) && !defined('HW_USE_JQUERY_FROM_CDN')) { $jquery_src = $url_base . '/' . $jquery_file_name; } elseif (defined('HW_JQUERY_CDN_SRC') && defined('HW_JQUERY_CDN_VERSION')) { $jquery_version = HW_JQUERY_CDN_VERSION; $jquery_src = sprintf(HW_JQUERY_CDN_SRC, $jquery_version); } else { $jquery_src = sprintf('https://code.jquery.com/jquery-%s.min.js', $jquery_version); } if (!empty($jquery_src)) { $wp_scripts->registered['jquery-core']->src = $jquery_src; $wp_scripts->registered['jquery-core']->ver = $jquery_version; $wp_scripts->registered['jquery']->deps = ['jquery-core']; $wp_scripts->registered['jquery']->ver = $jquery_version; } } } add_action('wp_enqueue_scripts', 'hw_enqueue_scripts_update_jquery', 50);
Look through the code and you’ll see that we check to see if something has tried to enqueue a script that depends on jquery-core (a standard script that ships with WordPress). If the script is queued then we look to see if we’ve got a file called jquery-3.5.1.min.js in the “jq” sub-folder in our custom child theme. Finally, if we haven’t got this file locally then we pull it from a CDN.
To reference this snippet, you’ll need to add something like this near the top of you custom child theme’s functions.php.
// Update the stock jQuery to something more up-to-date. require_once 'functions-jquery.php';
JavaScript Errors in the Browser Console
After applying the new version of jQuery, you might find some errors in the browser’s console. In particular if you’ve used the WordPress Plugin Boilerplate Editor to create a plugin. You might see something like “Uncaught TypeError: e.indexOf is not a function”…
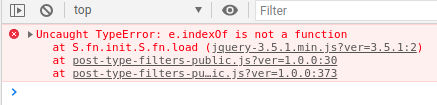
This is caused by the outdated initialisation code. In this case, the file is in post-type-filters-public.js, here:
* * Ideally, it is not considered best practise to attach more than a * single DOM-ready or window-load handler for a particular page. * Although scripts in the WordPress core, Plugins and Themes may be * practising this, we should strive to set a better example in our own work. */ $(window).load(function() { // ... // ...
All we have to do is change the handler so it’s like this:
* * Ideally, it is not considered best practise to attach more than a * single DOM-ready or window-load handler for a particular page. * Although scripts in the WordPress core, Plugins and Themes may be * practising this, we should strive to set a better example in our own work. */ $(window).on( 'load', function() { // ... // ...
Keep testing until your JavaScript console is clear of errors!
Test and Deploy your WordPress jQuery Version
Now all we need to do is run a couple of tests. Make sure you’re looking at a page on your WordPress site that’s referencing jQuery and then look through the page’s HTML source for “jquery-core”. It’ll be obvious if the new code is working because you’ll see the version is 3.5.1, instead of something older.
When you’re happy that it’s working, have a play with Lighthouse and check that your Best Practices is better than it was before, because you’ve closed-off the vulnerabilities caused by the older jQuery.
The final thing you need to do is choose…
- Self-host jQuery by putting jquery-3.5.1.min.js in a sub-folder called “jq” in your custom child theme.
- Pull jQuery from a CDN
Which of these options you go with is up to you, and depends on what sort of site traffic you’re expecting. Instructions for setting the CDN URL and jQuery version are in the comments at the top of functions-jquery.php.
Be sure to test your site thoroughly, as some plug-ins might be quite surprised to find a more recent version of jQuery than they expect. Generally speaking though, you should be fine…
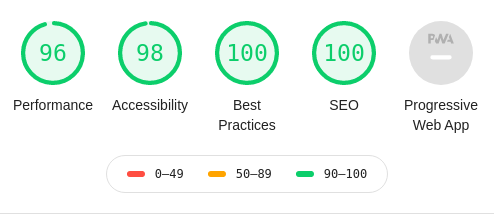
…and it makes your Lighthouse PageSpeed Insights report look cleaner 🙂
Not too shabby, and because we’ve kept this code in its own file, you can reuse functions-jquery.php in other projects quite easily 😀