Turn the regular WordPress gallery block into a series of CSS-animated link-tiles. It’s a fun way to add bling to your site without slowing down the page-load. We’ll set it up so all we need to do is add the “animated-gallery-links” class to any gallery block we want to animate.
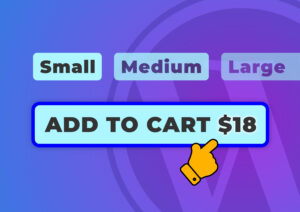
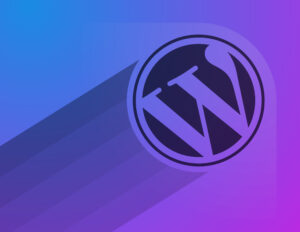
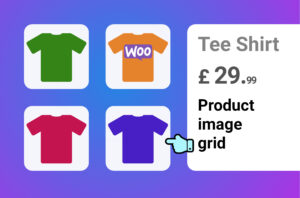
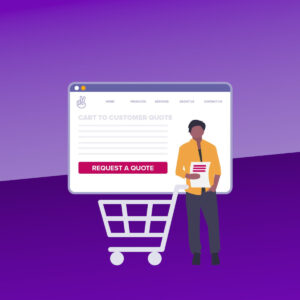
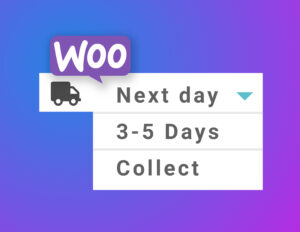
Scaffold the project
The only front-end asset we want in this project is a small CSS file, and we only it to be enqueued on pages where it’s needed. We could enqueue the CSS on every page-load, but that would be lazy and could impair the site’s performance. So we’ll create a small bit of PHP to detect when a gallery block is rendered, and if it has the “animated-gallery-links” CSS class, we’ll enqueue our CSS file.
In your custom child theme, create a file called “wpt-animated-gallery-links.php” and paste the following into it. There’s nothing complicated in here, it’s just a sequence of if...then...else
checks followed by a call to wp_enqueue_style() (if all the checks return true
).
<?php /** * WP Tutorials Animated Gallery Links (WPTAGL) * * https://wp-tutorials.tech/refine-wordpress/animated-wordpress-gallery-links/ * */ // Block direct access. defined('WPINC') || die(); // Add this CSS class to any WP Gallery block you want to animate. const WPTAGL_CLASS_NAME = 'animated-gallery-links'; /** * Detect when a standard WordPress gallery block is rendered with the * "animated-gallery-links" CSS class. * We don't modify the rendered content here - just enqueue our CSS file. */ function wptagl_render_block(string $block_content, array $block) { global $wptagl_are_assets_enqueued; if (!is_null($wptagl_are_assets_enqueued)) { // Our stylesheet has already been enqueued, // so we don't need to run any of these checks. } elseif (is_admin() || wp_doing_ajax()) { // We're not in the front-end. Don't do anything. } elseif ($block['blockName'] != 'core/gallery') { // This isn't a WP Gallery block. } elseif (!array_key_exists('attrs', $block)) { // The gallery block has no attributes. } elseif (!array_key_exists('className', $block['attrs'])) { // The className attribute is not specified. } elseif (empty($class_name = $block['attrs']['className'])) { // The className attribute is empty. } elseif (empty($gallery_classes = array_filter(explode(' ', $class_name)))) { // The className attribute is empty. } elseif (!in_array(WPTAGL_CLASS_NAME, $gallery_classes)) { // "animated-gallery-links" isn't in the list of CSS Classes. } else { $theme_version = wp_get_theme()->get('Version'); $base_uri = get_stylesheet_directory_uri(); wp_enqueue_style( 'animated-gallery-links', $base_uri . '/animated-gallery-links.css', null, // No dependencies $theme_version ); $wptagl_are_assets_enqueued = true; } return $block_content; } add_filter('render_block', 'wptagl_render_block', 10, 2);
Next, we need to edit your child theme’s functions.php and add the following couple of lines:
// WP Tutorials : Animated Gallery Links require_once dirname(__FILE__) . '/wpt-animated-gallery-links.php';
Finally, create a file in your child theme called “animated-gallery-links.css” and add the following, to get scaffold the styles.
/** * WP Tutorials Animated Gallery Links (WPTAGL) * * https://wp-tutorials.tech/refine-wordpress/animated-wordpress-gallery-links/ * */ .animated-gallery-links { margin-bottom: 1.5em; } .animated-gallery-links .wp-block-image { position: relative; overflow: hidden; } .animated-gallery-links .wp-block-image a { position: relative; display: block; }
Create a gallery block
Before we can add the actual CSS rules, create some content and add a gallery block with some images in it.
Select the gallery block (not any of the image blocks) and set the CSS Class to “animated-gallery-links”, under the “Advanced” tab.
For each image in the gallery, add a caption (to act as the link text) and set the target URLs for when each image is clicked.
Build the CSS animations
Now we can start on the fun stuff – creating the CSS animations in “animated-gallery-links.css”. Add the following to apply styles to the image captions, and animate them when the images are hovered.
/** * Configure and animate the image captions when hovered. */ .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image figcaption { background: transparent; font-size: 100%; font-weight: 900; text-shadow: -1px -1px 2px #000, 1px -1px 2px #000, -1px 1px 2px #000, 1px 1px 2px #000; position: absolute; text-align: left; transition: 0.3s bottom, 0.3s transform; padding-top: 0; padding-bottom: 0; padding-left: 1em; bottom: 1em; pointer-events: none; line-height: 1.3em; z-index: 20; } .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image:hover figcaption { bottom: 50%; transform: translateY(50%); }
All this does is set some styles for the figcaption
element, then changes its vertical position when the mouse hovers over the image.
To zoom/scale the image when hovered, add this chunk to the CSS file:
/** * Zoom in on the image when its hovered hover. */ .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image img { transition: 0.3s transform; } .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image:hover img { transform: scale(1.2); }
The following snippet adds a semi-transparent overlay when hovered.
/** * Create a totally transparent overlay for the image, then make it * semi-transparent when the image is hovered. */ .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image a::before { content: ' '; position: absolute; width: 100%; height: 100%; left: 0; top: 0; z-index: 10; transition: 0.3s opacity; background-color: black; opacity: 0.0; } .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image:hover a::before { opacity: 0.5; }
The final chunk adds an arrow that appears when the image is hovered. We’ve used Font Awesome 5 for the arrow, but you can use anything you want – or just don’t add this chunk if you don’t want the arrow to pop-up.
/** * Create an animated arrow that comes into view when the image is hovered. */ .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image a::after { font-family: 'Font Awesome 5 Free'; font-weight: 700; content: '\f061'; position: absolute; left: 0; bottom: 2em; color: #ADF5FF; opacity: 0.0; transition: 0.3s left, 0.3s opacity, 0.3s transform; transform: scale(0.5); z-index: 20; } .wp-block-gallery.has-nested-images.animated-gallery-links figure.wp-block-image:hover a::after { bottom: 2em; left: 1.2em; opacity: 1.0; transform: scale(1.5); }
And there we have it – a lightweight hack for the standard WordPress gallery block 😎👍